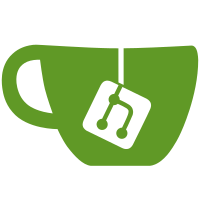
die()s in the code with Exception, making it possible to test properly. Fixed some outdated unit tests.
17346 lines
1.6 MiB
17346 lines
1.6 MiB
<?php
|
|
/**
|
|
* DO NOT EDIT: This file is automatically generated by configGenerator.php
|
|
*/
|
|
class configurationTest extends PHPUnit_Framework_TestCase
|
|
{
|
|
private static $pasteid = '5e9bc25c89fb3bf9';
|
|
|
|
private static $paste = array(
|
|
'data' => '{"iv":"EN39/wd5Nk8HAiSG2K5AsQ","v":1,"iter":1000,"ks":128,"ts":64,"mode":"ccm","adata":"","cipher":"aes","salt":"QKN1DBXe5PI","ct":"8hA83xDdXjD7K2qfmw5NdA"}',
|
|
'meta' => array(
|
|
'postdate' => 1344803344,
|
|
'opendiscussion' => true,
|
|
),
|
|
);
|
|
|
|
private $_model;
|
|
|
|
private $_conf;
|
|
|
|
public function setUp()
|
|
{
|
|
/* Setup Routine */
|
|
$this->_conf = PATH . 'cfg' . DIRECTORY_SEPARATOR . 'conf.ini';
|
|
if (!is_file($this->_conf . '.bak') && is_file($this->_conf))
|
|
rename($this->_conf, $this->_conf . '.bak');
|
|
|
|
$this->_model = zerobin_data::getInstance(array('dir' => PATH . 'data'));
|
|
serversalt::setPath(PATH . 'data');
|
|
$this->reset();
|
|
}
|
|
|
|
public function tearDown()
|
|
{
|
|
/* Tear Down Routine */
|
|
rename($this->_conf . '.bak', $this->_conf);
|
|
}
|
|
|
|
public function reset($configuration = array())
|
|
{
|
|
$_POST = array();
|
|
$_GET = array();
|
|
$_SERVER = array();
|
|
if ($this->_model->exists(self::$pasteid))
|
|
$this->_model->delete(self::$pasteid);
|
|
helper::createIniFile($this->_conf, $configuration);
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView0()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead0()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete0()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView1()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead1()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete1()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView2()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead2()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete2()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView3()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead3()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete3()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView4()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead4()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete4()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView5()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead5()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete5()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView6()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead6()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete6()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView7()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead7()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete7()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView8()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead8()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete8()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView9()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead9()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete9()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView10()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead10()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete10()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView11()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead11()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete11()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView12()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead12()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete12()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView13()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead13()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete13()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView14()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead14()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete14()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView15()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead15()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete15()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView16()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead16()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete16()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView17()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead17()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete17()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView18()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead18()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete18()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView19()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead19()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete19()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView20()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead20()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete20()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView21()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead21()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete21()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView22()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead22()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete22()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView23()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead23()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete23()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView24()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead24()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete24()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView25()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead25()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete25()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView26()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead26()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete26()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView27()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead27()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete27()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView28()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead28()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete28()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView29()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead29()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete29()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView30()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead30()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete30()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView31()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead31()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete31()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView32()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate32()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when sizelimit limit is not reached, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when sizelimit limit is not reached, paste exists after posting data');
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is disabled, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is disabled, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead32()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete32()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView33()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate33()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when sizelimit limit is not reached, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when sizelimit limit is not reached, paste exists after posting data');
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is disabled, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is disabled, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead33()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete33()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView34()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate34()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when sizelimit limit is not reached, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when sizelimit limit is not reached, paste exists after posting data');
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is disabled, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is disabled, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead34()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete34()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView35()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate35()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when sizelimit limit is not reached, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when sizelimit limit is not reached, paste exists after posting data');
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is disabled, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is disabled, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead35()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete35()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView36()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate36()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when sizelimit limit is not reached, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when sizelimit limit is not reached, paste exists after posting data');
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is disabled, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is disabled, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead36()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete36()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView37()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate37()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when sizelimit limit is not reached, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when sizelimit limit is not reached, paste exists after posting data');
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is disabled, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is disabled, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead37()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete37()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView38()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate38()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when sizelimit limit is not reached, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when sizelimit limit is not reached, paste exists after posting data');
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is disabled, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is disabled, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead38()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete38()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView39()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate39()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when sizelimit limit is not reached, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when sizelimit limit is not reached, paste exists after posting data');
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is disabled, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is disabled, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead39()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete39()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView40()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate40()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is disabled, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is disabled, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead40()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete40()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView41()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate41()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is disabled, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is disabled, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead41()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete41()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView42()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate42()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is disabled, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is disabled, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead42()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete42()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView43()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate43()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is disabled, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is disabled, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead43()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete43()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView44()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate44()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is disabled, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is disabled, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead44()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete44()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView45()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate45()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is disabled, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is disabled, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead45()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete45()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView46()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate46()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is disabled, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is disabled, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead46()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete46()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView47()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate47()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is disabled, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is disabled, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead47()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete47()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView48()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate48()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when sizelimit limit is not reached, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when sizelimit limit is not reached, paste exists after posting data');
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is disabled, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is disabled, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead48()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete48()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView49()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate49()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when sizelimit limit is not reached, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when sizelimit limit is not reached, paste exists after posting data');
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is disabled, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is disabled, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead49()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete49()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView50()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate50()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when sizelimit limit is not reached, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when sizelimit limit is not reached, paste exists after posting data');
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is disabled, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is disabled, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead50()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete50()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView51()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate51()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when sizelimit limit is not reached, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when sizelimit limit is not reached, paste exists after posting data');
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is disabled, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is disabled, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead51()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete51()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView52()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate52()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when sizelimit limit is not reached, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when sizelimit limit is not reached, paste exists after posting data');
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is disabled, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is disabled, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead52()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete52()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView53()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate53()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when sizelimit limit is not reached, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when sizelimit limit is not reached, paste exists after posting data');
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is disabled, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is disabled, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead53()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete53()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView54()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate54()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when sizelimit limit is not reached, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when sizelimit limit is not reached, paste exists after posting data');
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is disabled, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is disabled, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead54()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete54()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView55()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate55()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when sizelimit limit is not reached, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when sizelimit limit is not reached, paste exists after posting data');
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is disabled, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is disabled, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead55()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete55()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView56()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate56()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is disabled, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is disabled, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead56()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete56()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView57()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate57()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is disabled, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is disabled, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead57()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete57()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView58()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate58()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is disabled, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is disabled, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead58()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete58()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView59()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate59()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is disabled, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is disabled, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead59()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete59()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView60()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate60()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is disabled, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is disabled, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead60()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete60()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView61()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate61()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is disabled, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is disabled, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead61()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete61()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView62()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate62()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is disabled, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is disabled, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead62()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete62()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView63()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate63()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is disabled, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is disabled, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead63()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete63()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 0, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView64()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate64()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
$_POST["opendiscussion"] = "neither 1 nor 0";
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when discussions are enabled, but invalid flag posted, fail to create paste');
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'when discussions are enabled, but invalid flag posted, paste is not created');
|
|
$this->assertEquals(1, $response["status"], 'when sizelimit limit exceeded, fail to create paste');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead64()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete64()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView65()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead65()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete65()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView66()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate66()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when sizelimit limit exceeded, fail to create paste');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead66()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete66()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView67()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead67()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete67()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView68()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate68()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
$_POST["opendiscussion"] = "neither 1 nor 0";
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when discussions are enabled, but invalid flag posted, fail to create paste');
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'when discussions are enabled, but invalid flag posted, paste is not created');
|
|
$this->assertEquals(1, $response["status"], 'when sizelimit limit exceeded, fail to create paste');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead68()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete68()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView69()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead69()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete69()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView70()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate70()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when sizelimit limit exceeded, fail to create paste');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead70()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete70()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView71()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead71()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete71()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView72()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate72()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
$_POST["opendiscussion"] = "neither 1 nor 0";
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when discussions are enabled, but invalid flag posted, fail to create paste');
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'when discussions are enabled, but invalid flag posted, paste is not created');
|
|
$this->assertEquals(1, $response["status"], 'when sizelimit limit exceeded, fail to create paste');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead72()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete72()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView73()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead73()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete73()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView74()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate74()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when sizelimit limit exceeded, fail to create paste');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead74()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete74()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView75()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead75()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete75()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView76()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate76()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
$_POST["opendiscussion"] = "neither 1 nor 0";
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when discussions are enabled, but invalid flag posted, fail to create paste');
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'when discussions are enabled, but invalid flag posted, paste is not created');
|
|
$this->assertEquals(1, $response["status"], 'when sizelimit limit exceeded, fail to create paste');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead76()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete76()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView77()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead77()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete77()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView78()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate78()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when sizelimit limit exceeded, fail to create paste');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead78()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete78()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView79()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead79()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete79()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView80()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate80()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
$_POST["opendiscussion"] = "neither 1 nor 0";
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when discussions are enabled, but invalid flag posted, fail to create paste');
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'when discussions are enabled, but invalid flag posted, paste is not created');
|
|
$this->assertEquals(1, $response["status"], 'when sizelimit limit exceeded, fail to create paste');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead80()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete80()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView81()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead81()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete81()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView82()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate82()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when sizelimit limit exceeded, fail to create paste');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead82()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete82()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView83()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead83()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete83()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView84()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate84()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
$_POST["opendiscussion"] = "neither 1 nor 0";
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when discussions are enabled, but invalid flag posted, fail to create paste');
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'when discussions are enabled, but invalid flag posted, paste is not created');
|
|
$this->assertEquals(1, $response["status"], 'when sizelimit limit exceeded, fail to create paste');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead84()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete84()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView85()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead85()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete85()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView86()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate86()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when sizelimit limit exceeded, fail to create paste');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead86()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete86()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView87()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead87()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete87()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView88()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate88()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
$_POST["opendiscussion"] = "neither 1 nor 0";
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when discussions are enabled, but invalid flag posted, fail to create paste');
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'when discussions are enabled, but invalid flag posted, paste is not created');
|
|
$this->assertEquals(1, $response["status"], 'when sizelimit limit exceeded, fail to create paste');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead88()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete88()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView89()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead89()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete89()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView90()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate90()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when sizelimit limit exceeded, fail to create paste');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead90()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete90()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView91()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead91()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete91()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView92()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate92()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
$_POST["opendiscussion"] = "neither 1 nor 0";
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when discussions are enabled, but invalid flag posted, fail to create paste');
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'when discussions are enabled, but invalid flag posted, paste is not created');
|
|
$this->assertEquals(1, $response["status"], 'when sizelimit limit exceeded, fail to create paste');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead92()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete92()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView93()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead93()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete93()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView94()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate94()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when sizelimit limit exceeded, fail to create paste');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead94()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete94()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView95()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead95()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete95()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView96()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate96()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
$_POST["opendiscussion"] = "neither 1 nor 0";
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when discussions are enabled, but invalid flag posted, fail to create paste');
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'when discussions are enabled, but invalid flag posted, paste is not created');
|
|
$this->assertEquals(1, $response["status"], 'when sizelimit limit exceeded, fail to create paste');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead96()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete96()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView97()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead97()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete97()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView98()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate98()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when sizelimit limit exceeded, fail to create paste');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead98()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete98()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView99()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead99()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete99()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView100()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate100()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
$_POST["opendiscussion"] = "neither 1 nor 0";
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when discussions are enabled, but invalid flag posted, fail to create paste');
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'when discussions are enabled, but invalid flag posted, paste is not created');
|
|
$this->assertEquals(1, $response["status"], 'when sizelimit limit exceeded, fail to create paste');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead100()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete100()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView101()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead101()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete101()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView102()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate102()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when sizelimit limit exceeded, fail to create paste');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead102()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete102()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView103()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead103()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete103()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView104()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate104()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
$_POST["opendiscussion"] = "neither 1 nor 0";
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when discussions are enabled, but invalid flag posted, fail to create paste');
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'when discussions are enabled, but invalid flag posted, paste is not created');
|
|
$this->assertEquals(1, $response["status"], 'when sizelimit limit exceeded, fail to create paste');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead104()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete104()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView105()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead105()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete105()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView106()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate106()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when sizelimit limit exceeded, fail to create paste');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead106()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete106()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView107()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead107()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete107()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView108()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate108()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
$_POST["opendiscussion"] = "neither 1 nor 0";
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when discussions are enabled, but invalid flag posted, fail to create paste');
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'when discussions are enabled, but invalid flag posted, paste is not created');
|
|
$this->assertEquals(1, $response["status"], 'when sizelimit limit exceeded, fail to create paste');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead108()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete108()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView109()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead109()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete109()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView110()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate110()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when sizelimit limit exceeded, fail to create paste');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead110()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete110()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView111()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead111()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete111()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView112()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate112()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
$_POST["opendiscussion"] = "neither 1 nor 0";
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when discussions are enabled, but invalid flag posted, fail to create paste');
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'when discussions are enabled, but invalid flag posted, paste is not created');
|
|
$this->assertEquals(1, $response["status"], 'when sizelimit limit exceeded, fail to create paste');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead112()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete112()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView113()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead113()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete113()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView114()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate114()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when sizelimit limit exceeded, fail to create paste');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead114()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete114()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView115()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead115()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete115()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView116()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate116()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
$_POST["opendiscussion"] = "neither 1 nor 0";
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when discussions are enabled, but invalid flag posted, fail to create paste');
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'when discussions are enabled, but invalid flag posted, paste is not created');
|
|
$this->assertEquals(1, $response["status"], 'when sizelimit limit exceeded, fail to create paste');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead116()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete116()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView117()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead117()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete117()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView118()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate118()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when sizelimit limit exceeded, fail to create paste');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead118()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete118()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView119()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead119()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete119()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView120()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate120()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
$_POST["opendiscussion"] = "neither 1 nor 0";
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when discussions are enabled, but invalid flag posted, fail to create paste');
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'when discussions are enabled, but invalid flag posted, paste is not created');
|
|
$this->assertEquals(1, $response["status"], 'when sizelimit limit exceeded, fail to create paste');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead120()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete120()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView121()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead121()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete121()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView122()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate122()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when sizelimit limit exceeded, fail to create paste');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead122()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete122()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView123()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead123()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete123()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView124()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate124()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
$_POST["opendiscussion"] = "neither 1 nor 0";
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when discussions are enabled, but invalid flag posted, fail to create paste');
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'when discussions are enabled, but invalid flag posted, paste is not created');
|
|
$this->assertEquals(1, $response["status"], 'when sizelimit limit exceeded, fail to create paste');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead124()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete124()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView125()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead125()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete125()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView126()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate126()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when sizelimit limit exceeded, fail to create paste');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead126()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete126()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView127()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead127()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete127()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 10, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView128()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate128()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
$_POST["opendiscussion"] = "neither 1 nor 0";
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when discussions are enabled, but invalid flag posted, fail to create paste');
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'when discussions are enabled, but invalid flag posted, paste is not created');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead128()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete128()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView129()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate129()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is on and we wait, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is on and we wait, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead129()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete129()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView130()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate130()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead130()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete130()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView131()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate131()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is on and we wait, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is on and we wait, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead131()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete131()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView132()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate132()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
$_POST["opendiscussion"] = "neither 1 nor 0";
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when discussions are enabled, but invalid flag posted, fail to create paste');
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'when discussions are enabled, but invalid flag posted, paste is not created');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead132()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete132()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView133()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate133()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is on and we wait, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is on and we wait, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead133()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete133()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView134()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate134()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead134()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete134()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView135()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate135()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is on and we wait, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is on and we wait, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead135()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete135()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView136()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate136()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
$_POST["opendiscussion"] = "neither 1 nor 0";
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when discussions are enabled, but invalid flag posted, fail to create paste');
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'when discussions are enabled, but invalid flag posted, paste is not created');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead136()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete136()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView137()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate137()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is on and we wait, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is on and we wait, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead137()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete137()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView138()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate138()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead138()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete138()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView139()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate139()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is on and we wait, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is on and we wait, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead139()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete139()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView140()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate140()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
$_POST["opendiscussion"] = "neither 1 nor 0";
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when discussions are enabled, but invalid flag posted, fail to create paste');
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'when discussions are enabled, but invalid flag posted, paste is not created');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead140()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete140()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView141()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate141()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is on and we wait, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is on and we wait, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead141()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete141()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView142()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate142()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead142()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete142()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView143()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate143()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is on and we wait, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is on and we wait, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead143()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete143()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView144()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate144()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
$_POST["opendiscussion"] = "neither 1 nor 0";
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when discussions are enabled, but invalid flag posted, fail to create paste');
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'when discussions are enabled, but invalid flag posted, paste is not created');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead144()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete144()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView145()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate145()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is on and we wait, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is on and we wait, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead145()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete145()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView146()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate146()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead146()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete146()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView147()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate147()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is on and we wait, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is on and we wait, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead147()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete147()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView148()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate148()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
$_POST["opendiscussion"] = "neither 1 nor 0";
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when discussions are enabled, but invalid flag posted, fail to create paste');
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'when discussions are enabled, but invalid flag posted, paste is not created');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead148()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete148()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView149()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate149()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is on and we wait, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is on and we wait, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead149()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete149()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView150()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate150()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead150()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete150()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView151()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate151()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is on and we wait, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is on and we wait, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead151()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete151()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView152()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate152()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
$_POST["opendiscussion"] = "neither 1 nor 0";
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when discussions are enabled, but invalid flag posted, fail to create paste');
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'when discussions are enabled, but invalid flag posted, paste is not created');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead152()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete152()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView153()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate153()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is on and we wait, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is on and we wait, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead153()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete153()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView154()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate154()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead154()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete154()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView155()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate155()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is on and we wait, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is on and we wait, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead155()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete155()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView156()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate156()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
$_POST["opendiscussion"] = "neither 1 nor 0";
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when discussions are enabled, but invalid flag posted, fail to create paste');
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'when discussions are enabled, but invalid flag posted, paste is not created');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead156()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete156()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView157()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate157()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is on and we wait, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is on and we wait, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead157()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete157()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView158()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate158()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead158()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete158()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView159()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate159()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is on and we wait, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is on and we wait, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead159()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete159()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'page', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin\\.css#')), $content, 'outputs "page" stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'removes "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView160()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate160()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
$_POST["opendiscussion"] = "neither 1 nor 0";
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when discussions are enabled, but invalid flag posted, fail to create paste');
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'when discussions are enabled, but invalid flag posted, paste is not created');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead160()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete160()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView161()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate161()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is on and we wait, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is on and we wait, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead161()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete161()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView162()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate162()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead162()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete162()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView163()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate163()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is on and we wait, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is on and we wait, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead163()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete163()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView164()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate164()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
$_POST["opendiscussion"] = "neither 1 nor 0";
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when discussions are enabled, but invalid flag posted, fail to create paste');
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'when discussions are enabled, but invalid flag posted, paste is not created');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead164()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete164()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView165()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate165()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is on and we wait, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is on and we wait, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead165()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete165()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView166()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate166()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead166()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete166()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView167()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate167()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is on and we wait, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is on and we wait, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead167()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete167()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView168()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate168()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
$_POST["opendiscussion"] = "neither 1 nor 0";
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when discussions are enabled, but invalid flag posted, fail to create paste');
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'when discussions are enabled, but invalid flag posted, paste is not created');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead168()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete168()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView169()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate169()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is on and we wait, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is on and we wait, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead169()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete169()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView170()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate170()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead170()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete170()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView171()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate171()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is on and we wait, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is on and we wait, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead171()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete171()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView172()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate172()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
$_POST["opendiscussion"] = "neither 1 nor 0";
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when discussions are enabled, but invalid flag posted, fail to create paste');
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'when discussions are enabled, but invalid flag posted, paste is not created');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead172()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete172()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView173()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate173()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is on and we wait, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is on and we wait, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead173()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete173()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView174()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate174()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead174()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete174()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView175()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'preselects burn after reading option');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate175()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is on and we wait, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is on and we wait, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead175()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete175()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => true, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView176()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate176()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
$_POST["opendiscussion"] = "neither 1 nor 0";
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when discussions are enabled, but invalid flag posted, fail to create paste');
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'when discussions are enabled, but invalid flag posted, paste is not created');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead176()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete176()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView177()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate177()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is on and we wait, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is on and we wait, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead177()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete177()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView178()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate178()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead178()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete178()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView179()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate179()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is on and we wait, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is on and we wait, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead179()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete179()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'outputs prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView180()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate180()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
$_POST["opendiscussion"] = "neither 1 nor 0";
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when discussions are enabled, but invalid flag posted, fail to create paste');
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'when discussions are enabled, but invalid flag posted, paste is not created');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead180()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete180()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView181()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate181()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is on and we wait, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is on and we wait, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead181()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete181()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView182()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate182()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead182()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete182()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView183()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate183()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is on and we wait, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is on and we wait, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead183()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete183()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => 'sons-of-obsidian'), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView184()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate184()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
$_POST["opendiscussion"] = "neither 1 nor 0";
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when discussions are enabled, but invalid flag posted, fail to create paste');
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'when discussions are enabled, but invalid flag posted, paste is not created');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead184()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete184()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView185()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate185()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is on and we wait, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is on and we wait, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead185()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete185()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView186()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate186()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead186()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete186()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView187()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate187()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is on and we wait, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is on and we wait, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead187()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete187()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => true, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'outputs prettify stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'outputs prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView188()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate188()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
$_POST["opendiscussion"] = "neither 1 nor 0";
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when discussions are enabled, but invalid flag posted, fail to create paste');
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'when discussions are enabled, but invalid flag posted, paste is not created');
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead188()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete188()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView189()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate189()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is on and we wait, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is on and we wait, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead189()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete189()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => true, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertNotTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs enabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView190()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate190()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(1, $response["status"], 'when traffic limit is on and we do not wait, fail to create paste');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead190()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete190()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 10, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testView191()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('id' => 'burnafterreading', 'attributes' => array('checked' => 'checked')), $content, 'burn after reading option is unchecked');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testCreate191()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$_POST = self::$paste;
|
|
$_SERVER['REMOTE_ADDR'] = '::1';
|
|
sleep(3);
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$response = json_decode($content, true);
|
|
|
|
$this->assertEquals(0, $response["status"], 'when traffic limit is on and we wait, successfully create paste');
|
|
$this->assertTrue($this->_model->exists($response["id"]), 'when traffic limit is on and we wait, paste exists after posting data');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testRead191()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$_SERVER['QUERY_STRING'] = self::$pasteid;
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'cipherdata',
|
|
'content' => htmlspecialchars(json_encode(self::$paste), ENT_NOQUOTES)
|
|
),
|
|
$content,
|
|
'outputs data correctly'
|
|
);
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
/**
|
|
* @runInSeparateProcess
|
|
*/
|
|
public function testDelete191()
|
|
{
|
|
$this->reset(array('main' => array('opendiscussion' => false, 'syntaxhighlighting' => false, 'burnafterreadingselected' => false, 'sizelimit' => 2097152, 'template' => 'bootstrap', 'base64version' => '2.1.9', 'syntaxhighlightingtheme' => NULL), 'expire' => array('default' => '1month'), 'expire_options' => array('5min' => '300', '10min' => '600', '1hour' => '3600', '1day' => '86400', '1week' => '604800', '1month' => '2592000', '1year' => '31536000', 'never' => '0'), 'expire_labels' => array('5min' => '5 minutes', '10min' => '10 minutes', '1hour' => '1 hour', '1day' => '1 day', '1week' => '1 week', '1month' => '1 month', '1year' => '1 year', 'never' => 'Never'), 'traffic' => array('limit' => 2, 'dir' => '../data'), 'model' => array('class' => 'zerobin_data'), 'model_options' => array('dir' => '../data')));
|
|
$this->_model->create(self::$pasteid, self::$paste);
|
|
$this->assertTrue($this->_model->exists(self::$pasteid), 'paste exists before deleting data');
|
|
$_GET['pasteid'] = self::$pasteid;
|
|
$_GET['deletetoken'] = hash_hmac('sha1', self::$pasteid, serversalt::get());
|
|
ob_start();
|
|
new zerobin;
|
|
$content = ob_get_contents();
|
|
$this->assertTag(
|
|
array(
|
|
'id' => 'status',
|
|
'content' => 'Paste was properly deleted'
|
|
),
|
|
$content,
|
|
'outputs deleted status correctly'
|
|
);
|
|
$this->assertFalse($this->_model->exists(self::$pasteid), 'paste successfully deleted');
|
|
|
|
$this->assertTag(array('id' => 'opendiscussion', 'attributes' => array('disabled' => 'disabled')), $content, 'outputs disabled discussion correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/prettify\\.css#')), $content, 'removes prettify stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'script', 'attributes' => array('type' => 'text/javascript', 'src' => 'regexp:#js/prettify\\.js#')), $content, 'removes prettify javascript correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/prettify/sons-of-obsidian\\.css#')), $content, 'removes prettify theme stylesheet correctly');
|
|
$this->assertNotTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/zerobin.css#')), $content, 'removes "page" stylesheet correctly');
|
|
$this->assertTag(array('tag' => 'link', 'attributes' => array('type' => 'text/css', 'rel' => 'stylesheet', 'href' => 'regexp:#css/bootstrap/bootstrap-\\d[\\d\\.]+\\d\\.css#')), $content, 'outputs "bootstrap" stylesheet correctly');
|
|
}
|
|
|
|
}
|