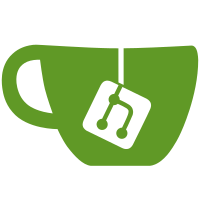
since they can see real JIDs, even if the room is semi-anonymous. Also made changes regarding programming style and conventions: - Pass in `this` to the template Recently I've started simplifying the `render` method by just passing `this` into the template and letting the template calculate what it needs from that. - Don't use `bind()` It's slow and arrow functions can be used instead. - Don't use an i18n string inside a tagged template literal Due to limitations with `xgettext` which cannot parse properly inside tagged template literals - Use snake-case for variables
49 lines
1.5 KiB
JavaScript
49 lines
1.5 KiB
JavaScript
import BaseModal from "plugins/modal/modal.js";
|
|
import tpl_occupant_modal from "./templates/occupant.js";
|
|
import { _converse, api } from "@converse/headless/core";
|
|
import { Model } from '@converse/skeletor/src/model.js';
|
|
|
|
export default class OccupantModal extends BaseModal {
|
|
|
|
initialize () {
|
|
super.initialize()
|
|
const model = this.model ?? this.message;
|
|
this.listenTo(model, 'change', () => this.render());
|
|
/**
|
|
* Triggered once the OccupantModal has been initialized
|
|
* @event _converse#occupantModalInitialized
|
|
* @type { Object }
|
|
* @example _converse.api.listen.on('occupantModalInitialized', data);
|
|
*/
|
|
api.trigger('occupantModalInitialized', { 'model': this.model, 'message': this.message });
|
|
}
|
|
|
|
getVcard () {
|
|
const model = this.model ?? this.message;
|
|
if (model.vcard) {
|
|
return model.vcard;
|
|
}
|
|
const jid = model?.get('jid') || model?.get('from');
|
|
return jid ? _converse.vcards.get(jid) : null;
|
|
}
|
|
|
|
renderModal () {
|
|
return tpl_occupant_modal(this);
|
|
}
|
|
|
|
getModalTitle () {
|
|
const model = this.model ?? this.message;
|
|
return model?.getDisplayName();
|
|
}
|
|
|
|
addToContacts () {
|
|
const model = this.model ?? this.message;
|
|
const jid = model.get('jid');
|
|
if (jid) {
|
|
api.modal.show('converse-add-contact-modal', {'model': new Model({ jid })});
|
|
}
|
|
}
|
|
}
|
|
|
|
api.elements.define('converse-muc-occupant-modal', OccupantModal);
|