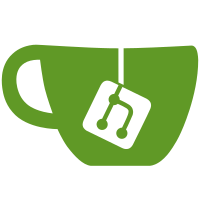
since they can see real JIDs, even if the room is semi-anonymous. Also made changes regarding programming style and conventions: - Pass in `this` to the template Recently I've started simplifying the `render` method by just passing `this` into the template and letting the template calculate what it needs from that. - Don't use `bind()` It's slow and arrow functions can be used instead. - Don't use an i18n string inside a tagged template literal Due to limitations with `xgettext` which cannot parse properly inside tagged template literals - Use snake-case for variables
23 lines
664 B
JavaScript
23 lines
664 B
JavaScript
import { generateFingerprint, getDevicesForContact, } from '../utils.js';
|
|
|
|
|
|
const ConverseMixins = {
|
|
|
|
async generateFingerprints (jid) {
|
|
const devices = await getDevicesForContact(jid);
|
|
return Promise.all(devices.map(d => generateFingerprint(d)));
|
|
},
|
|
|
|
getDeviceForContact (jid, device_id) {
|
|
return getDevicesForContact(jid).then(devices => devices.get(device_id));
|
|
},
|
|
|
|
async contactHasOMEMOSupport (jid) {
|
|
/* Checks whether the contact advertises any OMEMO-compatible devices. */
|
|
const devices = await getDevicesForContact(jid);
|
|
return devices.length > 0;
|
|
}
|
|
}
|
|
|
|
export default ConverseMixins;
|