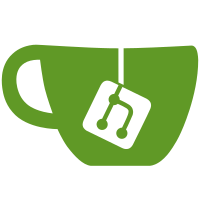
Also, moved locales.js to ./src folder. Add it as dependency to utils.js and remove it from the deps scripts
202 lines
8.0 KiB
JavaScript
202 lines
8.0 KiB
JavaScript
(function (root, factory) {
|
|
if (typeof define === 'function' && define.amd) {
|
|
define(["jquery", "converse-templates", "locales"], factory);
|
|
} else {
|
|
root.utils = factory(jQuery, templates);
|
|
}
|
|
}(this, function ($, templates, locales) {
|
|
"use strict";
|
|
|
|
var XFORM_TYPE_MAP = {
|
|
'text-private': 'password',
|
|
'text-single': 'textline',
|
|
'fixed': 'label',
|
|
'boolean': 'checkbox',
|
|
'hidden': 'hidden',
|
|
'jid-multi': 'textarea',
|
|
'list-single': 'dropdown',
|
|
'list-multi': 'dropdown'
|
|
};
|
|
|
|
$.expr[':'].emptyVal = function(obj){
|
|
return obj.value === '';
|
|
};
|
|
|
|
$.fn.hasScrollBar = function() {
|
|
if (!$.contains(document, this.get(0))) {
|
|
return false;
|
|
}
|
|
if(this.parent().height() < this.get(0).scrollHeight) {
|
|
return true;
|
|
}
|
|
return false;
|
|
};
|
|
|
|
$.fn.addHyperlinks = function () {
|
|
if (this.length > 0) {
|
|
this.each(function (i, obj) {
|
|
var x = $(obj).html();
|
|
var list = x.match(/\b(https?:\/\/|www\.|https?:\/\/www\.)[^\s<]{2,200}\b/g );
|
|
if (list) {
|
|
for (i=0; i<list.length; i++) {
|
|
var prot = list[i].indexOf('http://') === 0 || list[i].indexOf('https://') === 0 ? '' : 'http://';
|
|
var escaped_url = encodeURI(decodeURI(list[i])).replace(/[!'()]/g, escape).replace(/\*/g, "%2A");
|
|
x = x.replace(list[i], "<a target='_blank' href='" + prot + escaped_url + "'>"+ list[i] + "</a>" );
|
|
}
|
|
}
|
|
$(obj).html(x);
|
|
});
|
|
}
|
|
return this;
|
|
};
|
|
|
|
var utils = {
|
|
// Translation machinery
|
|
// ---------------------
|
|
__: function (str) {
|
|
// Translation factory
|
|
if (typeof this.i18n === "undefined") {
|
|
this.i18n = locales.en;
|
|
}
|
|
if (typeof this.i18n === "string") {
|
|
this.i18n = $.parseJSON(this.i18n);
|
|
}
|
|
if (typeof this.jed === "undefined") {
|
|
this.jed = new Jed(this.i18n);
|
|
}
|
|
var t = this.jed.translate(str);
|
|
if (arguments.length>1) {
|
|
return t.fetch.apply(t, [].slice.call(arguments,1));
|
|
} else {
|
|
return t.fetch();
|
|
}
|
|
},
|
|
|
|
___: function (str) {
|
|
/* XXX: This is part of a hack to get gettext to scan strings to be
|
|
* translated. Strings we cannot send to the function above because
|
|
* they require variable interpolation and we don't yet have the
|
|
* variables at scan time.
|
|
*
|
|
* See actionInfoMessages
|
|
*/
|
|
return str;
|
|
},
|
|
|
|
webForm2xForm: function (field) {
|
|
/* Takes an HTML DOM and turns it into an XForm field.
|
|
*
|
|
* Parameters:
|
|
* (DOMElement) field - the field to convert
|
|
*/
|
|
var $input = $(field), value;
|
|
if ($input.is('[type=checkbox]')) {
|
|
value = $input.is(':checked') && 1 || 0;
|
|
} else if ($input.is('textarea')) {
|
|
value = [];
|
|
var lines = $input.val().split('\n');
|
|
for( var vk=0; vk<lines.length; vk++) {
|
|
var val = $.trim(lines[vk]);
|
|
if (val === '')
|
|
continue;
|
|
value.push(val);
|
|
}
|
|
} else {
|
|
value = $input.val();
|
|
}
|
|
return $(templates.field({
|
|
name: $input.attr('name'),
|
|
value: value
|
|
}))[0];
|
|
},
|
|
|
|
xForm2webForm: function ($field, $stanza) {
|
|
/* Takes a field in XMPP XForm (XEP-004: Data Forms) format
|
|
* and turns it into a HTML DOM field.
|
|
*
|
|
* Parameters:
|
|
* (XMLElement) field - the field to convert
|
|
*/
|
|
|
|
// FIXME: take <required> into consideration
|
|
var options = [], j, $options, $values, value, values;
|
|
|
|
if ($field.attr('type') == 'list-single' || $field.attr('type') == 'list-multi') {
|
|
values = [];
|
|
$values = $field.children('value');
|
|
for (j=0; j<$values.length; j++) {
|
|
values.push($($values[j]).text());
|
|
}
|
|
$options = $field.children('option');
|
|
for (j=0; j<$options.length; j++) {
|
|
value = $($options[j]).find('value').text();
|
|
options.push(templates.select_option({
|
|
value: value,
|
|
label: $($options[j]).attr('label'),
|
|
selected: (values.indexOf(value) >= 0),
|
|
required: $field.find('required').length
|
|
}));
|
|
}
|
|
return templates.form_select({
|
|
name: $field.attr('var'),
|
|
label: $field.attr('label'),
|
|
options: options.join(''),
|
|
multiple: ($field.attr('type') == 'list-multi'),
|
|
required: $field.find('required').length
|
|
});
|
|
} else if ($field.attr('type') == 'fixed') {
|
|
return $('<p class="form-help">').text($field.find('value').text());
|
|
} else if ($field.attr('type') == 'jid-multi') {
|
|
return templates.form_textarea({
|
|
name: $field.attr('var'),
|
|
label: $field.attr('label') || '',
|
|
value: $field.find('value').text(),
|
|
required: $field.find('required').length
|
|
});
|
|
} else if ($field.attr('type') == 'boolean') {
|
|
return templates.form_checkbox({
|
|
name: $field.attr('var'),
|
|
type: XFORM_TYPE_MAP[$field.attr('type')],
|
|
label: $field.attr('label') || '',
|
|
checked: $field.find('value').text() === "1" && 'checked="1"' || '',
|
|
required: $field.find('required').length
|
|
});
|
|
} else if ($field.attr('type') && $field.attr('var') === 'username') {
|
|
return templates.form_username({
|
|
domain: ' @'+this.domain,
|
|
name: $field.attr('var'),
|
|
type: XFORM_TYPE_MAP[$field.attr('type')],
|
|
label: $field.attr('label') || '',
|
|
value: $field.find('value').text(),
|
|
required: $field.find('required').length
|
|
});
|
|
} else if ($field.attr('type')) {
|
|
return templates.form_input({
|
|
name: $field.attr('var'),
|
|
type: XFORM_TYPE_MAP[$field.attr('type')],
|
|
label: $field.attr('label') || '',
|
|
value: $field.find('value').text(),
|
|
required: $field.find('required').length
|
|
});
|
|
} else {
|
|
if ($field.attr('var') === 'ocr') { // Captcha
|
|
return _.reduce(_.map($field.find('uri'),
|
|
$.proxy(function (uri) {
|
|
return templates.form_captcha({
|
|
label: this.$field.attr('label'),
|
|
name: this.$field.attr('var'),
|
|
data: this.$stanza.find('data[cid="'+uri.textContent.replace(/^cid:/, '')+'"]').text(),
|
|
type: uri.getAttribute('type'),
|
|
required: this.$field.find('required').length
|
|
});
|
|
}, {'$stanza': $stanza, '$field': $field})
|
|
),
|
|
function (memo, num) { return memo + num; }, ''
|
|
);
|
|
}
|
|
}
|
|
}
|
|
};
|
|
return utils;
|
|
}));
|