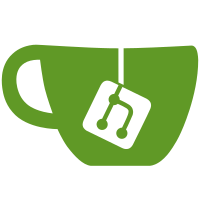
Had to make various other changes due to incompatibilities. - Use the new `lit` package instead of `lit-html` or `lit-element` - Drop `haunted` since it breaks the rules by specifying `type: module` but then doesn't import with file extensions - Use Sass Dart instead of node-sass (fixes #2445) - Upgrade Karma
44 lines
1.3 KiB
JavaScript
44 lines
1.3 KiB
JavaScript
import 'shared/components/icons.js';
|
|
import xss from 'xss/dist/xss';
|
|
import { CustomElement } from 'shared/components/element.js';
|
|
import { api } from '@converse/headless/core';
|
|
import { html } from 'lit';
|
|
import { unsafeHTML } from 'lit/directives/unsafe-html.js';
|
|
|
|
|
|
export default class ChatHelp extends CustomElement {
|
|
|
|
static get properties () {
|
|
return {
|
|
chat_type: { type: String },
|
|
messages: { type: Array },
|
|
model: { type: Object },
|
|
type: { type: String }
|
|
}
|
|
}
|
|
|
|
render () {
|
|
const isodate = (new Date()).toISOString();
|
|
return [
|
|
html`<converse-icon class="fas fa-times close-chat-help"
|
|
@click=${this.close}
|
|
path-prefix="${api.settings.get("assets_path")}"
|
|
size="1em"></converse-icon>`,
|
|
...this.messages.map(m => this.renderHelpMessage({
|
|
isodate,
|
|
'markup': xss.filterXSS(m, {'whiteList': {'strong': []}})
|
|
}))
|
|
];
|
|
}
|
|
|
|
close () {
|
|
this.model.set({'show_help_messages': false});
|
|
}
|
|
|
|
renderHelpMessage (o) {
|
|
return html`<div class="message chat-${this.type}" data-isodate="${o.isodate}">${unsafeHTML(o.markup)}</div>`;
|
|
}
|
|
}
|
|
|
|
api.elements.define('converse-chat-help', ChatHelp);
|