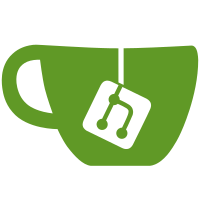
* (Android) Get rid of double bangs by using Kotlin view binding Instead of holding a nullable reference to the WebView, we are now accessing the WebView using the view binding utility of Kotlin's Android Extensions. Further reading: https://kotlinlang.org/docs/tutorials/android-plugin.html * (Android) Enable WebView debugging in debug builds This enables debugging the app's WebView using Chrome's DevTools. https://developers.google.com/web/tools/chrome-devtools/remote-debugging/webviews * (Android) Make MainActivity.kt adhere to common Kotlin conventions * (Android) Update dependencies and improve formatting of Gradle files This updates the Kotlin plugin to 1.3.21 and the Gradle plugin to 3.3.2 * (Android) Remove unnecessary ConstraintLayout container Layout files should generally have as few nested layers as possible, because every layer affects the performance. * (Android) Use JSONObject class to construct a JSON string It is way safer to construct a JSON string using classes that are meant for doing that, instead of concatenating raw strings. * (Android) Suppress JavaScript lint warning * (Android) Use Kotlin string templates instead of concatenating strings * (Android) Add missing SuppressLint import
42 lines
1.5 KiB
Groovy
42 lines
1.5 KiB
Groovy
apply plugin: 'com.android.application'
|
|
apply plugin: 'kotlin-android'
|
|
apply plugin: 'kotlin-android-extensions'
|
|
|
|
android {
|
|
compileSdkVersion 27
|
|
defaultConfig {
|
|
applicationId "org.mozilla.firefoxsend"
|
|
minSdkVersion 26
|
|
targetSdkVersion 27
|
|
versionCode 1
|
|
versionName "1.0"
|
|
testInstrumentationRunner "android.support.test.runner.AndroidJUnitRunner"
|
|
}
|
|
buildTypes {
|
|
release {
|
|
minifyEnabled false
|
|
proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro'
|
|
}
|
|
}
|
|
}
|
|
|
|
dependencies {
|
|
implementation fileTree(dir: 'libs', include: ['*.jar'])
|
|
implementation"org.jetbrains.kotlin:kotlin-stdlib-jdk7:$kotlin_version"
|
|
implementation 'com.android.support:appcompat-v7:27.1.1'
|
|
implementation 'com.android.support.constraint:constraint-layout:1.1.3'
|
|
testImplementation 'junit:junit:4.12'
|
|
androidTestImplementation 'com.android.support.test:runner:1.0.2'
|
|
androidTestImplementation 'com.android.support.test.espresso:espresso-core:3.0.2'
|
|
implementation 'com.github.delight-im:Android-AdvancedWebView:v3.0.0'
|
|
implementation "org.mozilla.components:service-firefox-accounts:$android_components_version"
|
|
}
|
|
|
|
task generateAndLinkBundle(type: Exec, description: 'Generate the android.js bundle and link it into the assets directory') {
|
|
commandLine './buildAssets.sh'
|
|
}
|
|
|
|
tasks.withType(JavaCompile) {
|
|
compileTask -> compileTask.dependsOn generateAndLinkBundle
|
|
}
|