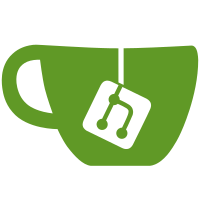
Also: * Refactor interacting with a remote event so that you can interact with a remote group as well * Add a setting for group admins to pick between an open and invite-only group * Fix new groups without posts/todos/resources/events/conversations URL set * Repair local groups that haven't got their posts/todos/resources/events/conversations URL set * Add a scheduled job to refresh remote groups every hour * Add a user setting to pick when to receive notifications when there's new members to approve (will be used when this feature is available) * Fix pagination for members Signed-off-by: Thomas Citharel <tcit@tcit.fr>
95 lines
2.8 KiB
Vue
95 lines
2.8 KiB
Vue
<docs>
|
|
```vue
|
|
<participant-card :participant="{ actor: { preferredUsername: 'user1', name: 'someoneIDontLike' }, role: 'REJECTED' }" />
|
|
```
|
|
|
|
```vue
|
|
<participant-card :participant="{ actor: { preferredUsername: 'user2', name: 'someoneWhoWillWait' }, role: 'NOT_APPROVED' }" />
|
|
```
|
|
|
|
```vue
|
|
<participant-card :participant="{ actor: { preferredUsername: 'user3', name: 'a_participant' }, role: 'PARTICIPANT' }" />
|
|
```
|
|
|
|
```vue
|
|
<participant-card :participant="{ actor: { preferredUsername: 'me', name: 'myself' }, role: 'CREATOR' }" />
|
|
```
|
|
</docs>
|
|
<template>
|
|
<article class="card">
|
|
<div class="card-content">
|
|
<div class="media">
|
|
<div class="media-left" v-if="participant.actor.avatar">
|
|
<figure class="image is-48x48">
|
|
<img :src="participant.actor.avatar.url" />
|
|
</figure>
|
|
</div>
|
|
<div class="media-content">
|
|
<span ref="title">{{ actorDisplayName }}</span
|
|
><br />
|
|
<small class="has-text-grey" v-if="participant.actor.domain"
|
|
>@{{ participant.actor.preferredUsername }}@{{ participant.actor.domain }}</small
|
|
>
|
|
<small class="has-text-grey" v-else>@{{ participant.actor.preferredUsername }}</small>
|
|
</div>
|
|
</div>
|
|
</div>
|
|
<footer class="card-footer">
|
|
<b-button
|
|
v-if="[ParticipantRole.NOT_APPROVED, ParticipantRole.REJECTED].includes(participant.role)"
|
|
@click="accept(participant)"
|
|
type="is-success"
|
|
class="card-footer-item"
|
|
>{{ $t("Approve") }}</b-button
|
|
>
|
|
<b-button
|
|
v-if="participant.role === ParticipantRole.NOT_APPROVED"
|
|
@click="reject(participant)"
|
|
type="is-danger"
|
|
class="card-footer-item"
|
|
>{{ $t("Reject") }}</b-button
|
|
>
|
|
<b-button
|
|
v-if="participant.role === ParticipantRole.PARTICIPANT"
|
|
@click="exclude(participant)"
|
|
type="is-danger"
|
|
class="card-footer-item"
|
|
>{{ $t("Exclude") }}</b-button
|
|
>
|
|
<span v-if="participant.role === ParticipantRole.CREATOR" class="card-footer-item">{{
|
|
$t("Creator")
|
|
}}</span>
|
|
</footer>
|
|
</article>
|
|
</template>
|
|
|
|
<script lang="ts">
|
|
import { Component, Prop, Vue } from "vue-property-decorator";
|
|
import { IParticipant, ParticipantRole } from "../../types/participant.model";
|
|
import { IPerson, Person } from "../../types/actor";
|
|
|
|
@Component
|
|
export default class ParticipantCard extends Vue {
|
|
@Prop({ required: true }) participant!: IParticipant;
|
|
|
|
@Prop({ type: Function }) accept!: Function;
|
|
|
|
@Prop({ type: Function }) reject!: Function;
|
|
|
|
@Prop({ type: Function }) exclude!: Function;
|
|
|
|
ParticipantRole = ParticipantRole;
|
|
|
|
get actorDisplayName(): string {
|
|
const actor = new Person(this.participant.actor as IPerson);
|
|
return actor.displayName();
|
|
}
|
|
}
|
|
</script>
|
|
|
|
<style lang="scss">
|
|
.card-footer-item {
|
|
height: $control-height;
|
|
}
|
|
</style>
|